Sometimes, you might want to get user IP address using PHP in your PHP application for authorization or logging purposes. This article will show you how to do so.
Quick solution
The quickest way to get user IP address using PHP is by getting the $_SERVER['REMOTE_ADDR']
variable:
$userIP = $_SERVER['REMOTE_ADDR'];
This will return the current user IP address (your IP, when viewing the page yourself).
However, getting user IP address using this variable isn’t always reliable. Except the case that user spoofing their IP address (by using VPN for example), the IP address might not be accurate if your server is behind a WAF (Web Application Firewall, such as CloudFlare).
The below section will further guide you on multiple cases to get the accurate user IP address in PHP.
Tutorial StartsTo get user IP address using PHP
Get the right IP variable
As the intro, the basic $_SERVER
variable for getting user IP address is $_SERVER['REMOTE_ADDR']
.
However, if your server is behind a WAF (Web Application Firewall), the variable will be different. There are different type of WAF, but we will point out the commonly used WAF, CloudFlare and Imperva (Incapsula).
- For CloudFlare, the correct variable of user IP address is
$_SERVER['HTTP_CF_CONNECTING_IP']
. - For Imperva (Incapsula), the variable is
$_SERVER['HTTP_INCAP_CLIENT_IP']
.
After figuring out the correct IP variable, we can get user IP address using multiple methods.
Get user IP address methods
Using PHPInfo
First, create a PHP file with the following line:
<?php phpinfo();
Save the file, open it on the browser, and lookup the IP variable mentioned in the previous section.
Example for the web server using Imperva Web Application Firewall:
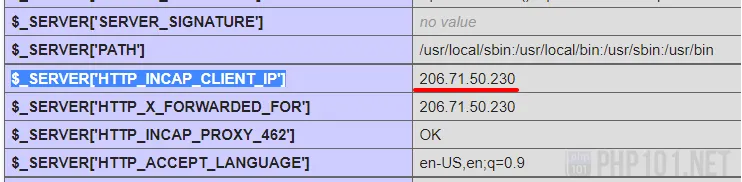
Echoing $_SERVER
variable
To view user IP address by echoing the $_SERVER
variable, simply echo the proper IP variable mentioned in the above section to view user IP address:
<?php
// Native IP address
echo $_SERVER['REMOTE_ADDR'];
// CloudFlare
echo $_SERVER['HTTP_CF_CONNECTING_IP'];
// Imperva (Incapsula)
echo $_SERVER['HTTP_INCAP_CLIENT_IP'];
Final thoughts
With the correct $_SERVER
variable, it is easy to get user IP address using PHP. While it’s not always 100% correct, especially when the user intended to spoof their IP address, it’s accurate enough for general use cases.